giga::core::FileDownloader Class Reference
#include <FileDownloader.h>
Inheritance diagram for giga::core::FileDownloader:
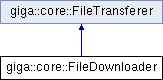
Classes | |
struct | Result |
Public Types | |
enum | Policy { Policy::override, Policy::overrideNewerSize, Policy::ignore, Policy::rename } |
enum | Action { fileOverriden, fileIgnored, fileRenamed, fileDownloaded } |
![]() | |
enum | State { pending, started, paused, canceled, error } |
Public Member Functions | |
FileDownloader (const boost::filesystem::path &folderDest, const Node &node, const Application &app, pplx::cancellation_token_source cts=pplx::cancellation_token_source{}, Policy policy=Policy::ignore) | |
Construct a FileDownloader. More... | |
FileDownloader (FileDownloader &&other) | |
FileDownloader (const FileDownloader &)=delete | |
FileDownloader & | operator= (const FileDownloader &)=delete |
FileDownloader & | operator= (FileDownloader &&)=delete |
const pplx::task< Result > & | task () const |
Gets the task managing the download. Make sure it has been started first (see FileTransferer::start() ) More... | |
const boost::filesystem::path & | downloadingFile () const |
const boost::filesystem::path & | destinationFile () const |
FileTransferer::Progress | progress () const override |
const boost::filesystem::path & | filename () const override |
![]() | |
FileTransferer (pplx::cancellation_token_source cts) | |
FileTransferer (FileTransferer &&other) | |
FileTransferer (const FileTransferer &)=delete | |
FileTransferer & | operator= (const FileTransferer &)=delete |
FileTransferer & | operator= (FileTransferer &&)=delete |
void | start () |
Start a transfer. | |
void | pause () |
Pause the current transfer (see resume() ) | |
void | resume () |
Resume the current transfer (see pause() ) | |
void | cancel () |
Cancel a started upload. The task managing the transfer will most probably throw an exception. | |
void | setError (const std::string &error) |
State | state () const |
void | limitRate (uint64_t rate) |
Limit the rate of the transfer. More... | |
Protected Member Functions | |
void | doStart () override |
Additional Inherited Members | |
![]() | |
State | _state |
std::unique_ptr < details::CurlProgress > | _progress |
std::mutex | _mut |
pplx::cancellation_token_source | _cts |
utility::string_t | _error |
Detailed Description
Download a file
Member Enumeration Documentation
|
strong |
Constructor & Destructor Documentation
|
explicit |
Construct a FileDownloader.
- Parameters
-
folderDest the folder in which we want to put the downloaded node node the node to download app the authenticated application cts a cancel token to use for canceling the download task policy what to do if a file with the same name already exists
Member Function Documentation
const path & giga::core::FileDownloader::destinationFile | ( | ) | const |
At the end of the download process, the file will be here
const path & giga::core::FileDownloader::downloadingFile | ( | ) | const |
During the download process, we use this temporary file
const pplx::task< FileDownloader::Result > & giga::core::FileDownloader::task | ( | ) | const |
Gets the task managing the download. Make sure it has been started first (see FileTransferer::start()
)
The documentation for this class was generated from the following files:
- /home/thomas/code/GiGaSdk/src/giga/core/FileDownloader.h
- /home/thomas/code/GiGaSdk/src/giga/core/FileDownloader.cpp